How to use Redis in Node.js
Redis is one of many technologies that can help you improve the performance of web applications. Get acquainted with TipsMake.com with Redis and learn how to optimize your Node applications with this powerful datastore.
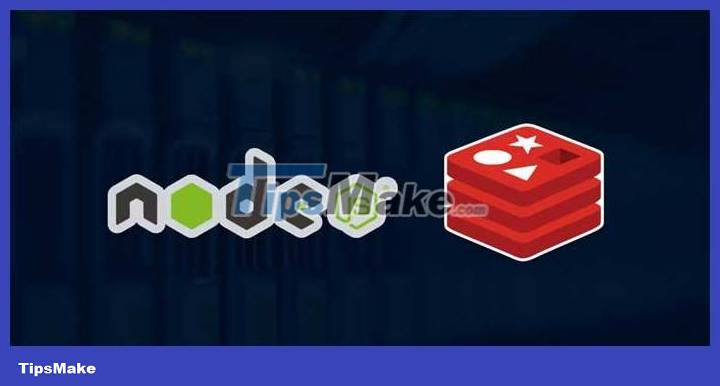
When building a web app, you need to make sure it's scalable and functional. For this to work, you need to take into account a number of principles and concepts. Redis offers great features in enhancing the performance of any web-based application.
So what exactly is Redis , how can you use Redis with Node.js web application? Let's find out together!
What is Redis, when to use Redis?
Redis is an open source, in-memory key-value store. Redis is a flexible technology that can act as a job queue, database, cache, session manager, message relay module, etc.
Redis's combination of speed, flexibility, and ease of use makes it a very valuable tool in the software developer ecosystem.
When should Redis be used?
Redis is suitable for many tasks, but you should use it with caution, preferably only when you really need it.
You should consider using Redis in the following situations:
- Caching : Redis is the most popular way to implement caches that help prevent database load and improve overall performance.
- Session Management : Store and manage user sessions for easy authentication and state management with Redis.
- Temporary data storage : Redis allows you to store data and delete it automatically after a certain period of time. This makes it a good tool for storing temporary data like JSON web tokens, job queues, etc.
Other scenarios you should consider using Redis include implementing message brokers, optimizing database operations, and even storing and accessing efficient data structures like lists, sets, and hashes.
How to use Redis with Node.js
Node.js is a popular technology for building scalable and efficient server-side apps. You can use it in conjunction with assistive technologies like Redis for maximum performance and scalability.
Redis supports several languages. You can use it to store data in PHP and there are excellent Redis clients for Golang. The two most popular Redis clients for Node.js are node-redis and ioredis .
Connecting to Redis from Node.js
To get started, you must have Redis installed on your computer and set up a Node.js development environment.
You need to install your preferred Redis client in your project to be able to connect to Redis. Depending on the configuration, run one of the commands below to install the Redis client.
npm install redis --save npm install ioredis --save
The first command is to install node-redis , the second is to install ioredis .
To configure the freshly installed client, make sure Redis is running. You can do this by starting redis-cli in the terminal:
The command ping ping server to confirm it is running, and a PONG response in the CLI means that the server is still up and running. Now create the redis.js file in your Node project and write the following code in it to configure Redis:
const redis = require("redis") // khởi tạo bằng cách dùng cấu hình mặc định const RedisClient = redis.createClient() const connectRedis = async () => { // kết nối với redis await RedisClient.connect() // xử lý lỗi RedisClient.on('error', (err) => { console.error(`An error occurred with Redis: ${err}`) }) console.log('Redis connected successfully.') }
The above code initializes a new Redis client in this application. The redis package's createClient function allows you to create a client using selected parameters.
You can specify host, port, username, password or connection string or use default values. For example, node-redis will start running on localhost, on port 6379 by default.
Here are a few ways you can use the createClient function :
// dùng chuỗi kết nối const RedisClient = redis.createClient("rediss://127.0.0.1:6379") // dùng đối tượng tham số const RedisClient = redis.createClient({ socket: { host: "127.0.0.1", port: "6379" }, username: "your redis username", password: "your password" })
Implement Basic Redis Operations in Node.js
The following code connects Redis using the connectRedis function above and demonstrates some of the basic operations you can perform in Redis from Node.
const testRedis = async () => { await connectRedis() // Đặt và truy xuất một giá trị await RedisClient.set("name", "Timmy") const getCommand = await RedisClient.get("name") console.log(getCommand) // Xóa các giá trị ngay lập tức hoặc lên lịch hết hạn chúng // trong vài giây await RedisClient.del("name"); await RedisClient.setEx("location", 60, "Lagos") // Đẩy vào phía trước hoặc sau danh sách await RedisClient.lPush("days", "monday") await RedisClient.rPush("days", "tuesday") await RedisClient.lPush("days", "wednesday") const daysArray = await RedisClient.lRange("days", 0, -1) // prints [ 'wednesday', 'monday', 'tuesday' ] console.log(daysArray) // Loại bỏ mục ở sau hoặc trước danh sách await RedisClient.rPop("days") await RedisClient.lPop("days") const newDaysArray = await RedisClient.lRange("days", 0, -1) // prints [ 'monday' ] console.log(newDaysArray) } testRedis()
Notice how async and await are used here. This is because all functions run unevenly and return promises, so if you need immediate results, you need to await them.
The above code shows just some of the basic operations that you can perform in Redis from a Node.js application. There are many other commands and concepts that are worth learning about.
The Redis Commands section of the README found in the node-redis GitHub repository is a great place to find all the other commands and know how they work.
Above is how to use Redis to increase the performance of the application created with Node.js . Hope the article is useful to you.
You should read it
- Schema validation in Node.js using Joi
- Instructions for installing Node.js
- Event Loop in Node.js
- Concept of Buffer in Node.js
- Read the File record in Node.js
- Utility Module in Node.js
- What is Node.js?
- REPL Terminal in Node.js
- NPM in Node.js
- 10 things not to do when running Node.js application
- Hello World program in Node.js
- Global objects in Node.js
Maybe you are interested
AI Product Images Are Everywhere: How to Shop Safely? Instructions for using Signal messaging application on phone, PC What is Text Blaze? How does it work on Chrome? How to fix print streaks, wrong colors on Canon color inkjet printers 'Detox' Facebook in a scientific way Pomodoro 'tomato' method: Working focused, highly effective without fatigue