Class Inheritance in Python (Part 2)
In the previous part, I mentioned the basic properties of classes in Python. In this part, I will mention the advanced properties and characteristics of classes.
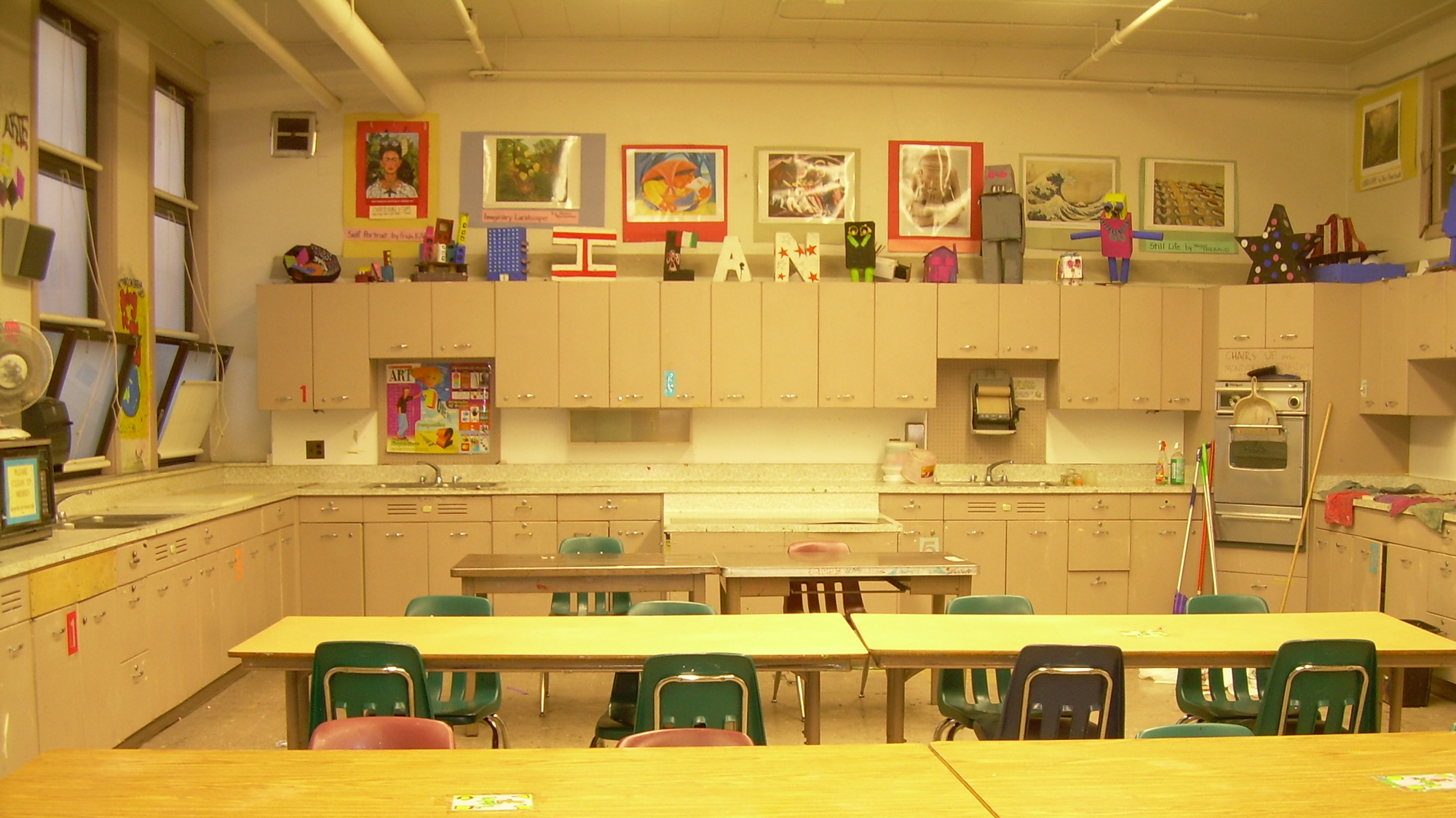
In the previous part, I mentioned the basic properties of classes in Python. In this part, I will mention the advanced properties and characteristics of classes.
I. Multi-inheritance
Unlike Java — a language that only allows inheritance of a single class for a class, Python allows us to inherit multiple classes for a class. For example:
The result of Child.bases in this case is:
II. Find attributes with inheritance
Logically, the process of finding attributes is as follows:
- First, look up attributes in local dict
- If not, continue looking in the class dict
- If it still doesn't exist, look in the classes in mro.
I will clarify the last point in the following parts of the article.
III. MRO
MRO stands for Method Resolution Order. It is an inheritance chain that Python calculates and stores in the MRO attribute in the class. As mentioned above, when looking for attributes, Python will go through the elements in MRO in turn.
To view the MRO of a class, use the syntax ".__mro__". For example:
Output:
Python uses cooperative multiple inheritance to enforce some rules about class order:
- Children are always checked before parents (children classes are always checked before parents classes) Parents (if multiple) are always checked in the order listed (parents classes are always checked in the order listed)
- As in the above example, after checking at E, class D will be checked as D is listed first among the two base classes. Then, B — parent class of D will be checked. Finally, class C and its parent — A will be checked.
To understand better, please try to brainstorm and explain the MRO of class E below:
This algorithm is called "C3 Linearization Algorithm" but to keep it simple and easy to understand, imagine the order of escape when an incident like a house fire or a ship sinking occurs: "Children first, followed by parents".
IV. Examples of multi-inheritance
super() will call the next class of the current class in the MRO
For example:
NoisyPerson's MRO:
Try calling the talks method of the NoisyPerson instance:
Output:
When the girl's talk() method is called, since NoisyPerson itself does not have this method, it will look for it in the next class in the MRO, Noisy. Noisy has a talk() method that will be executed. super().talk() will look for the talk() method of the next class in the MRO - Person (return 'alo alo'). This string 'alo alo' will be upper()ed and returned. Therefore the output returned is "ALO ALO"
Now what if we swap the two parent classes?
Rerun the talks method
Output:
Here is NoisyPerson's MRO now:
We see that the Person class is checked before Noisy, while Person has a talks() method so it will be executed.
V. Conclusion
Through the two parts on the topic of class, I hope you have more knowledge about class and can apply it to your work. Please look forward to the next articles on the topic of Python on the tech blog TipsMake!
You should read it
- Storage class in C / C ++
- Class selector in CSS
- Pseudo-Class in CSS
- Class (Class) in C #
- How to Crash a Class in College
- Storage class in C programming
- Interface in C ++ (Abstract class)
- How To Make The Best Class Assignments?
- How to Write a Class in Python
- Contructor and Destructor in C ++
- Learn Class and Object in Python
- Class member functions in C ++
Maybe you are interested
NASA's space telescope finds three new planets with rare features Not any organization, but these families are quietly controlling the world Explore the rainbow-colored village of fever in Indonesia Maintaining these 9 habits, you don't want to get old Looking at the earwax, you will know right away if you are susceptible to armpit odor. Discover human limits through 5 senses