Merge Linked List Problem
Continuing the Linked List topic in the Algorithm & Data Structure series, today I will introduce to you the Merge Linked List problem. In this article, I will give 2 solutions to the problem and analyze the space and time complexity.
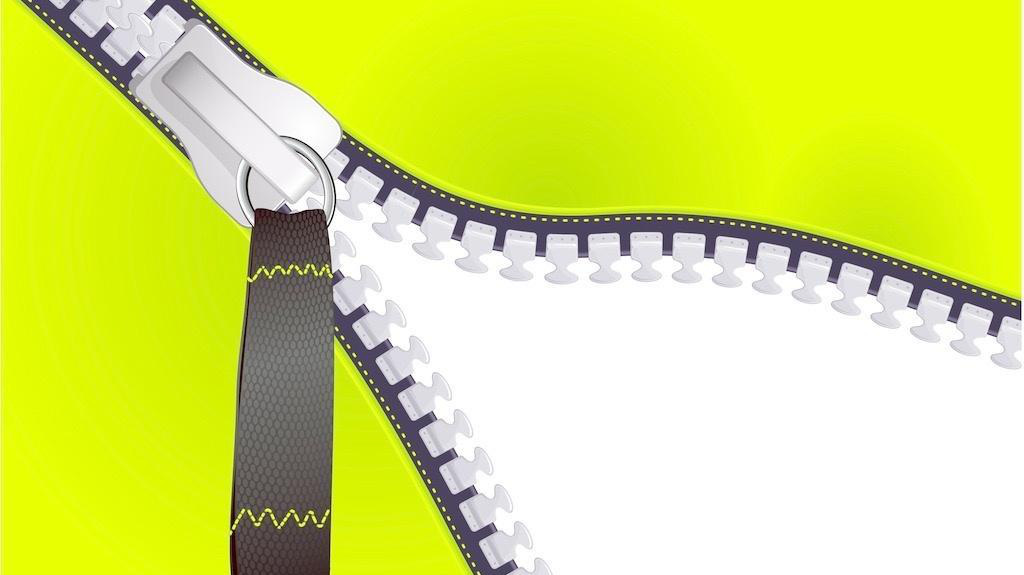
Introduce
Continuing the Linked List topic in the Algorithm & Data Structure series, today I will introduce to you the Merge Linked List problem. In this article, I will give 2 ways to solve the problem and analyze the space and time complexity. I will assume that you already know about the linked list data structure when reading this article. If not, you can read the document "Basic linked list" of Stanford University, USA here.
Topic
Write a function that takes in 2 heads of two sorted Singly linked lists. Write a function that merges the two linked lists and returns the head of the merged sorted linked list.
Input:
Output:
In the next section, I will give two ways to solve this problem.
Solution 1: use list as intermediary
Similar to the previous linked list problems, we can solve this problem through lists and then reconstruct a linked list. Here is my description:
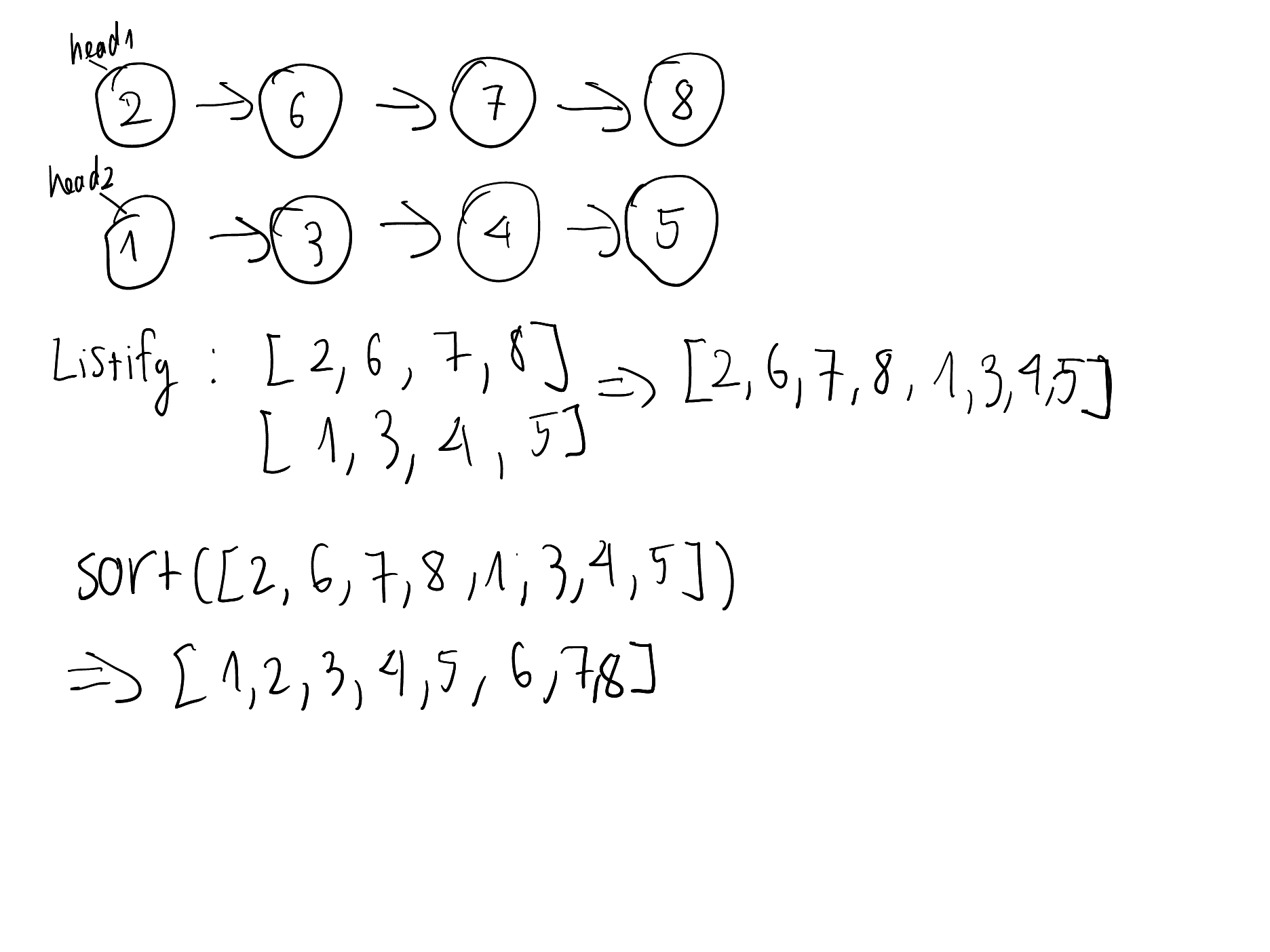
The detailed steps are as follows:
- First we convert two linked lists into two lists
- Then we merge those two lists together.
- Newly created sort list
- Construct new linked list based on sorted list
My solution to this approach:
Space and time complexity analysis:
Note: m and n are the lengths of the two linked lists respectively.
Space complexity is O(n + m)
The space complexity to listify two linked lists is O(n + m). The space complexity to construct a linked list from the new list is O(n + m). Therefore, the total space complexity is O(n + m).
Time complexity is O((n+m)log(n+m))
Time complexity to listify two linked lists is O(n+m) Time complexity to sort the list is O((n + m)log(n + m)) Time complexity to construct the linked list from the new list is O(n+m) Therefore, total time complexity is O((n + m)log(n + m)
Solution 2: Merge in place
Instead of going through the list like the above method, this way we will merge two linked lists right on the selected one. Here is my description:
The detailed steps are as follows:
- Choose the head with the smaller value as the center - where the remaining linked list nodes will be inserted
- Use two pointers if to insert nodes into the central linked list in order.
- Loop through all nodes in both linked lists
My solution is as follows:
Space and time complexity analysis:
Note: m and n are the lengths of the two linked lists respectively.
Space complexity is O(1)
- Since the entire algorithm is merged in place, the space complexity constant - O(1)
Time complexity is O(n+m)
- The time complexity to iterate through each element of two linked lists is O(n + m)
III. Conclusion
The above two solutions are not all the ways to merge two linked lists, but they are two quite typical ways for this classic problem. Hopefully this blog post can help you in some way. If you find the blog post interesting, please share it so that more people know about it. And look forward to more of my blog posts on the topic of Data Structure & Algorithm on TipsMake's tech blog!
You should read it
- Reverse linked list
- Data structure of double linked list
- Linked list data structure (Linked List)
- Data Link List structure (Circular Linked List)
- Shift linked list problem
- Microsoft adds a page to manage devices sharing the same user account on Windows 11
- The To-do List is good, the Done List is very good, but the Do-Not-Do List is much better
- How to create a drop list in Excel 2016
- Work with lists in PowerPoint 2016
- How to Simplify Your To-Do List
- PowerPoint 2016: Working with lists in PowerPoint
- List () function in Python
Maybe you are interested
What is ftdibus.sys on Windows? Why does it disable Memory Integrity? The Cryptojacking problem in Southeast Asia fell 78% after INTERPOL intervened Collection of the best fun puzzles to play in the Mid-Autumn Festival The standard 'no need to adjust' makeup steps for dry skin Decode the phenomenon of strange dry, bubbly sand like boiling water NASA discovered water in planetary Neptune's atmosphere