How to implement Infinite Scroll in web application
Infinite scroll - Infinite scrolling allows content to load continuously as the user moves down the page, unlike the click-to-load method of traditional page alignment. This feature can provide a smoother experience, especially on mobile devices.

Let's explore with TipsMake how to set up simple Infinite scroll using HTML, CSS and JavaScript !
Set up the frontend
Start with basic HTML structure to display content. Here is an example:
Page scrolls infinitely
This page contains a string of placeholder images and references two sources: a CSS file and a JavaScript file.
CSS styles for scrollable content
To display the placeholder image in a grid, add the following CSS to the style.css file :
* { margin: 0; padding: 0; box-sizing: border-box; } html { font-size: 62.5%; } body { font-family: Cambria, Times, "Times New Roman", serif; } h1 { text-align: center; font-size: 5rem; padding: 2rem; } img { width: 100%; display: block; } .products__list { display: flex; flex-wrap: wrap; gap: 2rem; justify-content: center; } .products__list > * { width: calc(33% - 2rem); } .loading-indicator { display: none; position: absolute; bottom: 30px; left: 50%; background: #333; padding: 1rem 2rem; color: #fff; border-radius: 10px; transform: translateX(-50%); }
Currently, your page will look like this:
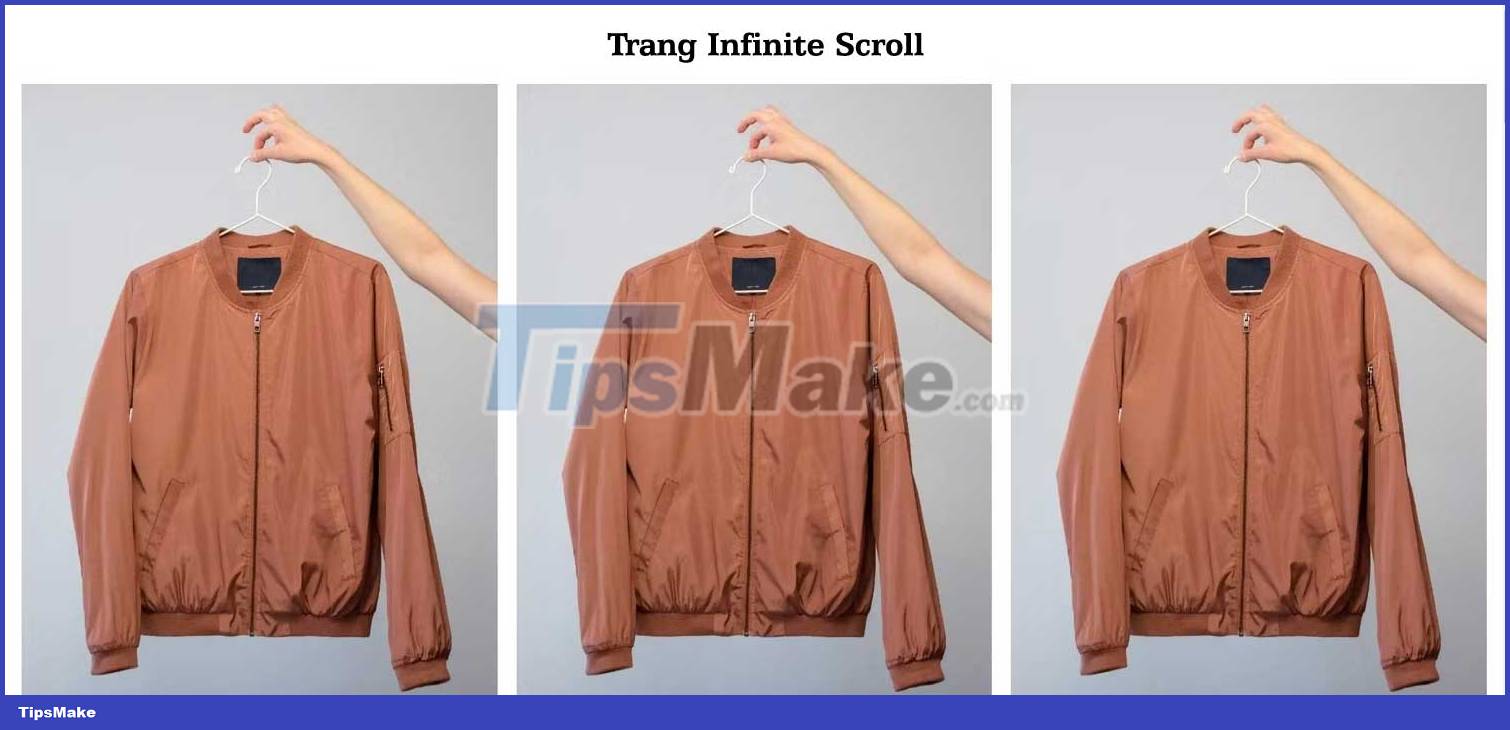
Core implementation with JS
Edit script.js. To implement infinite scroll, you need to detect when the user has scrolled near the bottom of the container or content page.
"use strict"; window.addEventListener("scroll", () => { if ( window.scrollY + window.innerHeight >= document.documentElement.scrollHeight - 100 ) { // Người dùng ở gần dưới cùng, tìm nạp nhiều nội dung hơn fetchMoreContent(); } });
Then create a function to fetch more placeholder data.
async function fetchMoreContent() { try { let response = await fetch("https://fakestoreapi.com/products?limit=3"); if (!response.ok) { throw new Error("Network response was not ok"); } let data = await response.json(); console.log(data); } catch (error) { console.error("There was a problem fetching new content:", error); } finally { console.log("Fetch function fired"); } }
For this project, you can use the API from fakestoreapi .
To confirm data is fetched on scroll, look at the console:
You will see the data fetched multiple times as you scroll. It may be a factor affecting the performance of the device. To avoid this, create an initial data fetch state:
let isFetching = false;
Then, edit the fetch function to only find data after a previously completed process.
async function fetchMoreContent() { if (isFetching) return; // Thoát nếu tìm nạp dữ liệu đã xong isFetching = true; // Đặt flag sang true try { let response = await fetch("https://fakestoreapi.com/products?limit=3"); if (!response.ok) { throw new Error("Network response was not ok"); } let data = await response.json(); } catch (error) { console.error("There was a problem fetching new content:", error); } finally { console.log("Fetch function fired"); isFetching = false; // Reset flag sang false } }
Show new content
To show new content when the user scrolls down the page, create a function that appends the images to the main container.
First, choose the main component:
const productsList = document.querySelector(".products__list");
Then create a function that appends content:
function displayNewContent(data) { data.forEach((item) => { const imgElement = document.createElement("img"); imgElement.src = item.image; imgElement.alt = item.title; productsList.appendChild(imgElement); // Append to productsList container }); }
Finally, edit the fetch function and pass the fetched data to the append function.
async function fetchMoreContent() { if (isFetching) return; isFetching = true; try { let response = await fetch("https://fakestoreapi.com/products?limit=3"); if (!response.ok) { throw new Error("Network response was not ok"); } let data = await response.json(); displayNewContent(data); } catch (error) { console.error("There was a problem fetching new content:", error); } finally { console.log("Fetch function fired"); isFetching = false; } }
That's it, infinite scrolling will now work.
Enhance infinite scrolling
To enhance user experience, you can display a loading indicator when fetching new content. Start by adding this HTML.
Loading.
Then select the loading component.
const loadingIndicator = document.querySelector(".loading-indicator");
Finally, create two functions that enable/disable loading indicator visibility.
function showLoadingIndicator() { loadingIndicator.style.display = "block"; console.log("Loading."); } function hideLoadingIndicator() { loadingIndicator.style.display = "none"; console.log("Finished loading."); }
Then add them to the fetch function.
async function fetchMoreContent() { if (isFetching) return; // Exit if already fetching isFetching = true; showLoadingIndicator(); // Show loader try { let response = await fetch("https://fakestoreapi.com/products?limit=3"); if (!response.ok) { throw new Error("Network response was not ok"); } let data = await response.json(); displayNewContent(data); } catch (error) { console.error("There was a problem fetching new content:", error); } finally { console.log("Fetch function fired"); hideLoadingIndicator(); // Hide loader isFetching = false; } }
Result:
Things to note when using infinite scroll:
- Don't fetch too many items at once as it may reduce browser performance.
- Instead of fetching content immediately after detecting a scroll event, use a debug function to delay the fetch a bit. This can prevent excessive network queries.
- Not all users like infinite scrolling. Provide the option to use a pagination component if desired.
If there isn't much content left to load, notify the user instead of constantly trying to fetch more data.
You should read it
- How to Implement Infinite Scrolling in Vue
- What is Infinite Scrolling and how does it work?
- How to implement infinite scrolling and pagination with Next.js and TanStack Query
- How to Implement Smooth Scrolling in JavaScript
- Infinite Galaxy game tips for newbies
- MSI launches new gaming desktop series with powerful configuration and impressive design
- How to take scrolling, long screen screenshots in Windows
- How to turn on and turn off smooth scrolling on Opera
- Instructions for scrolling TikTok videos automatically
- Why are websites scrolling vertically and not horizontal scrolling?
- How to enable two-finger scrolling on Windows laptop
- How to add a scrolling camera to PyGame
Maybe you are interested
Characters who could be the final villains in One Piece How to safely continue using a Smart TV that no longer receives updates 4 Easiest and Quickest Ways to Share Wi-Fi Password Instructions for viewing match history in Lien Quan Mobile What does astrology say about each generation? 3 ways to check the graphics card model on Windows 11