Iterator object in Python
Iterators are objects that allow us to take each of its elements, this action can be repeated. In this article, Quantrimang will discuss with you how the iterator works in Python and how you can build your own iterator using __iter__ and __next__ methods.
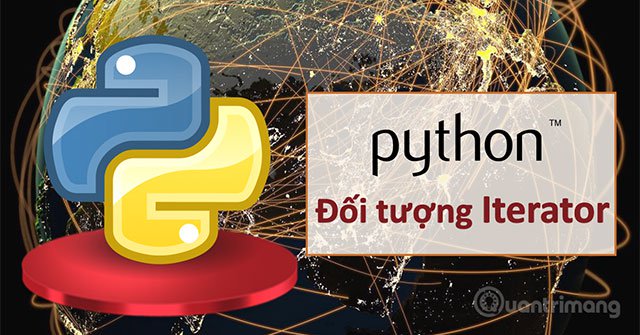
What is an iterator in Python?
Iterator is everywhere in Python, inside loops, comprehension, generator .
It is simply objects that allow us to retrieve each element, whenever you use loops or techniques to get the value of an element group at a given time.
Technically, Python in Python must implement two special methods: __iter __ () and __next __ (), collectively referred to as the iterator protocol (Iterator Protocol).
- The __iter__ method returns the iterator object itself. This method is required to install both "iterable" and iterator objects in order to use for and print statements.
- The __next__ method returns the next element. If there are no more elements, a StopIteration error will occur.

Iterable objects are an object after using methods that will return an iterator, such as String, List, and Tuple.
Iter () is a built-in function in Python that takes the input as an iterable object and returns an iterator.
# Khai bao mot list
my_list = [4, 7, 0, 3]
# lay mot iterator bang cach su dung iter()
my_iter = iter(my_list)
## su dung next()
#prints 4
print(next(my_iter))
#prints 7
print(next(my_iter))
## next(obj) chinh la obj.__next__()
#prints 0
print(my_iter.__next__())
#prints 3
print(my_iter.__next__())
## Xay ra loi StopIteration vi het gia tri tra ve
next(my_iter)
Run the program, the result is:
4
7
0
3
Traceback (most recent call last):
File "", line 24, in
next(my_iter)
StopIteration
A similar way to return this result is to use a for loop .
>>> for element in my_list:
. print(element)
.
4
7
0
3
How the loop works
As we see in the above example, the for loop can be repeated automatically through the use of the list.
In fact, the for loop can be repeated on any iterable. Let's take a closer look at how a for loop is implemented in Python.
for element in iterable:
# do something with element
Made similar to:
# iter_obj là một iterator object tạo từ iterable
iter_obj = iter(iterable)
# vòng lặp
while True:
try:
# sử dụng next
element = next(iter_obj)
except StopIteration:
# nếu xảy ra lỗi StopIteration thì vòng lặp sẽ được break ra ngoài
break
In this example, inside the for loop we create an iterator object called iter_obj by calling iter () on iterable.
And as you can see, the for loop here is an infinite while loop.Next () inside the loop retrieves the elements to execute commands in for loop. When all values are taken, the StopIteration exception will be generated and the loop will end.
Build iterator iterator in Python
We can build the iterator as a class. Building an iterator is easy in Python, we only need to implement __iter __ () and __next __ () methods.
class PowTwo:
def __init__(self, max = 0):
self.max = max
def __iter__(self):
self.n = 0
return self
def __next__(self):
if self.n <= self.max:
result = 2 ** self.n
self.n += 1
return result
else:
raise StopIteration
The __iter__ method will cause the object to become an iterable object.
The return value of __iter__ is an iterator. It needs a __next__ method and returns StopIteration if there are no more tests.
Create an iterator and run the program as follows:
>>> a = PowTwo(4)
>>> i = iter(a)
>>> next(i)
1
>>> next(i)
2
>>> next(i)
4
>>> next(i)
8
>>> next(i)
16
>>> next(i)
Traceback (most recent call last):
.
StopIteration
We can also use a for loop to iterator iterators
>>> for i in PowTwo(5):
. print(i)
.
1
2
4
8
16
32
Iterator iterates infinitely in Python
Not all iterator objects will be called all elements and end when there are no elements left. There are some iterator instances that will loop infinitely. Examples are as follows:
>>> int()
0
>>> inf = iter(int,1)
>>> next(inf)
0
>>> next(inf)
0
We can see that the int () function always returns 0. Therefore, passing it in iter (int, 1) will return an iterator until the value returns to 1. This is never happens and this is an infinite loop iterator. You need to pay attention when handling in such cases.
Alternatively, you can also build an infinite loop iterator. The following example will loop indefinitely and return odd numbers because there are no stop conditions.
class InfIter:
def __iter__(self):
self.num = 1
return self
def __next__(self):
num = self.num
self.num += 2
return num
Run the program:
>>> a = iter(InfIter())
>>> next(a)
1
>>> next(a)
3
>>> next(a)
5
>>> next(a)
7
The advantage of using iterator iterators is that they save resources. As shown above, you can get all odd numbers without storing on the entire digital system in memory.
See more:
- Object-oriented programming in Python
- Inheritance (Inheritance) in Python
- The loop technique in Python
Previous lesson: Operator overloading in Python
You should read it
- Generator in Python
- Zip () function in Python
- The next () function in Python
- The reversed () function in Python
- All () function in Python
- The function frozenset () in Python
- Enumerate () function in Python
- The iter () function in Python
- The object () function in Python
- Max () function in Python
- Object-oriented programming in Python
- Learn Class and Object in Python
Maybe you are interested
If money is keeping you up at night, these financial habits can help you get out of this problem. How to Communicate Well With People from Other Cultures 7 signs that you still haven't earned enough money to spend 5 things should be a little more and 5 things should be invested more 18 things that financial adults don't usually do 11 bad habits that prevent you from getting out of debt