The reversed () function in Python
The reversed () function is one of the built-in functions in Python, used to reverse the original string returning the iterator. What syntax is the reversed () function, and what are the parameters, let's find out in this article Quantrimang.
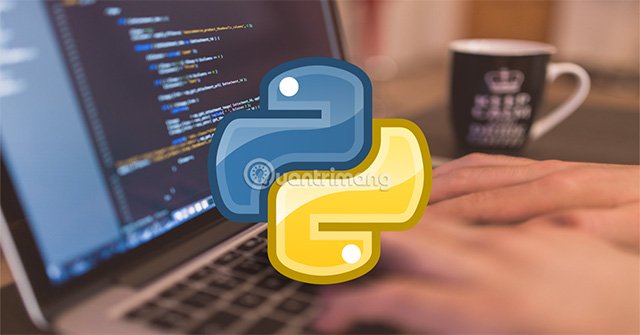
The syntax of the reversed () function in Python
reversed(seq)
Parameters of the reversed () function:
Reversed () has only one parameter:
seq
: the string you want to reverse.
Strings are objects that support string protocols like __len __ () and __getitem __ () methods. For example: tuple, string, list, range .
Can use reversed () with any object implementing __reverse __ ()
Return value from (reversed)
The reversed () function allows us to process the items in the reverse order of the original string, receive the string and return an iterator with the reversed value of the original string passed.
Example 1: Using reversed () with string, tuple, list and range
print( reversed([44, 11, -90, 55, 3]) ) # với string seq_string = 'Python' print(list(reversed(seq_string))) # với tuple seq_tuple = ('P', 'y', 't', 'h', 'o', 'n') print(list(reversed(seq_tuple))) # với range seq_range = range(5, 9) print(list(reversed(seq_range))) # với list seq_list = [1, 2, 4, 3, 5] print(list(reversed(seq_list)))
Running the program, the result is returned:
['n', 'o', 'h', 't', 'y', 'P'] ['n', 'o', 'h', 't', 'y', 'P'] [8, 7, 6, 5] [5, 3, 4, 2, 1]
In the above example, we are converting the result of reversed () to list using the list () function.
Example 2: reversed () with custom objects
class Vowels: vowels = ['a', 'e', 'i', 'o', 'u'] def __reversed__(self): return reversed(self.vowels) v = Vowels() print(list(reversed(v)))
Running the program, the result is returned:
['u', 'o', 'i', 'e', 'a']
See also: Python built-in functions.
You should read it
- The map () function in Python
- The oct () function in Python
- Max () function in Python
- The next () function in Python
- The function set () in Python
- The ord () function in Python
- The slice () function in Python
- The function id () in Python
- The pow () function in Python
- Min () function in Python
- Sum () function in Python
- The float () function in Python