The staticmethod () function in Python
The function staticmethod () is considered un-Pythonic (Python's unorthodox language). In newer versions of Python, you should use the @staticmethod decorator instead.
The syntax of @staticmethod is:
@staticmethod def func(args, .)
The syntax of the staticmethod () function in Python
The function staticmethod () is determined by the following syntax:
staticmethod(function)
Parameters of the staticmethod () function
The staticmethod () function has a single parameter:
- Function: The function needs to be converted into a static method
The return value of the staticmethod () function
The staticmethod () function returns a static method for a parameter function that you include.
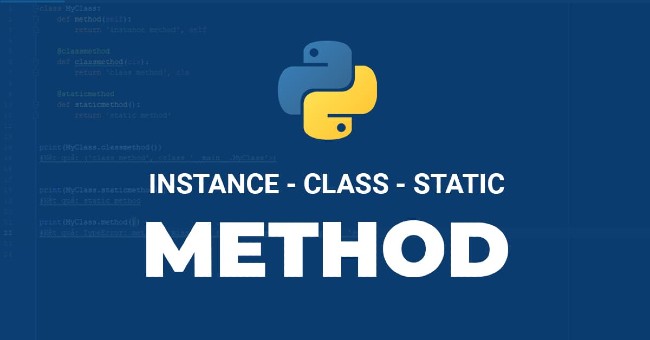
What is static method?
Static methods, like class methods, are methods that are associated with a class and not its objects. Static methods have the advantage of not depending on the state of the object.
The difference between a static method and a class method is:
The static method knows nothing about the class and only processes parameters. Meanwhile, the class method is always based on the class because its parameter is always the class itself.
Both static methods and class methods can be called by both classes and objects.
Class.staticmethodFunc() or even Class().staticmethodFunc()
Example 1: Create a static method using the staticmethod () function
class Tipsmake: def addnumbers(x, y): return x + y # make static method add numbers Tipsmake.addnumbers = staticmethod(Tipsmake.addnumbers) print('The total is:', Tipsmake.addnumbers(5, 10))
When running the program, the result is:
The total is : 15
When should we use static methods?
1. Group utility functions into a class
The applicability of static methods is quite limited because, like the class method or any method within the class, they cannot access the properties of the class itself.
However, when you need a utility function that does not access any properties of a class but makes sense that it belongs to that class, we will use the static method.
Example 2: Creating a utility function using static methods
class Dates: def __init__(self, date): self.date = date def getDate(self): return self.date @staticmethod def toDashDate(date): return date.replace("/", "-") date = Dates("15-12-2016") dateFromDB = "15/12/2016" dateWithDash = Dates.toDashDate(dateFromDB) if(date.getDate() == dateWithDash): print("Match") else: print("Not Match")
Running the program results in:
Match
Here, we have the Dates class which only works with the date data using dashes to indicate. However, in the old data, all dates use slashes to indicate.
To convert a date using a slash to a date using a hyphen, we create a utility function toDashDate inside Dates .
It is a static method because it does not need to access any of the properties of Dates and requires only input parameters.
We can also create toDashDate outside of the class but since it only processes data related to dates, leaving it in the Dates class is a logical choice, helping the code to be cleaner.
2. There is only one executable
Static methods are used when we do not want a subclass in a class to change / override a special implementation of a method.
Example 3: How does inheritance work with static methods?
class Dates: def __init__(self, date): self.date = date def getDate(self): return self.date @staticmethod def toDashDate(date): return date.replace("/", "-") class DatesWithSlashes(Dates): def getDate(self): return Dates.toDashDate(self.date) date = Dates("15-12-2016") dateFromDB = DatesWithSlashes("15/12/2016") if(date.getDate() == dateFromDB.getDate()): print("Match") else: print("Not Match")
Result
Match
Here, we do not want the subclasses DatesWithSlashes to override static utility method toDashDate because it is only used once, for example changing the date from the form using the slash to the form of dash.
We can easily use the static method to get more benefits by overriding the getDate () method in the subclass so it works well with the DatesWithSlashes class .
- Python functions built in
- If, if . else, if . elif . else statements in Python
- Data types in Python: string, number, list, tuple, set and dictionary
You should read it
- Max () function in Python
- The ord () function in Python
- Int () function in Python
- Zip () function in Python
- The function id () in Python
- The oct () function in Python
- Hex () function in Python
- The function dir () in Python
- The map () function in Python
- The next () function in Python
- The function set () in Python
- Help () function in Python
Maybe you are interested
iPhone XS Max and 6s Plus officially become 'classic' devices
How to decorate a beautiful 20/11 board for the classroom, 20/11 board decoration sample
Some classic Fallout games are free on the Epic Games Store
The 5 best apps and websites to watch classic movies
4 classic Linux tools that have better modern alternatives
Restore classic File Explorer with ribbon in Windows 11