The hash () function in Python
In Python, the hash () function will return the hash value of the object if any. The hash function generally takes any content input and then uses algorithms to generate output of a specific length.
In this article we will learn the hash () function, the syntax and usage of the hash () function in Python.
The syntax of the hash () function in Python:
hash()
The hash parameter ()
The hash () function takes a single parameter:
- Object: Is anything to get the hash value (integer, string, real number .)
The value returned from the hash () function
The hash () function returns the hash value of the object (if any).
If the object has a custom __hash __ () function, the hash () function will cut the return value into Py_ssize_t size.
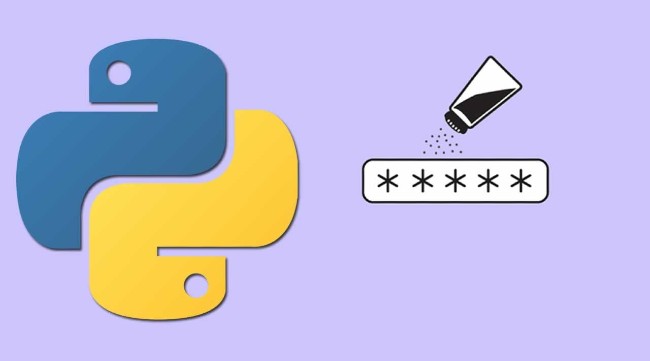
How does the hash () function in Python work?
Listing 1. How hash () works in Python
#hash cho số nguyên không thay đổi print('Hash của 181 là:', hash (181)) #hash cho số thập phân print('Hash cho 181.23 là:',hash(181.23)) #hash cho chuỗi print('Hash cho TipsMake.com là:', hash('TipsMake.com'))
When running the program, the result returned is:
Hash của 181 là: 181 Hash cho 181.23 là: 530343892119126197 Hash cho TipsMake.com là: 3327670722527760259
Listing 2. hash () for invariant object tuple
The hash () function only works with immutable objects like tuples. Tuple in Python is a data type used to store objects unchanged later (like constants).
#tuple của TipsMake TipsMake = ('Q', 'u', 'a', 'n') print('Hash của TipsMake là:', hash(TipsMake))
When running the program, the result returned is:
Hash của TipsMake là: 4475739666350434463
How does the hash () function handle custom objects?
As mentioned above, the hash () function calls an internal __hash __ () function. Therefore, any object can override __hash __ () to get a custom hash value.
But to implement the hash function correctly, __hash __ () must always return an integer result. And, both __eq __ () and __hash __ () functions must be implemented.
The following is the case for applying hashes to custom objects
Cases where applying hashes to custom objects __eq_ () __hash __ () Definition Definition (by default) Determining (by default) If left, all of the compared objects are not equal to each other ( except if they are themselves) (If Variable) Determined Not Defined The process of applying a collection of hashable values requires that the hash values of the key be immutable. If __eq __ () is not specified, __hash __ () will not be defined Determined Unknown Class fields cannot use a hashable value collection. __hash __ () will be set to None. Increase the TypeError exception if trying to redeploy the hash Determine Controlled by parent class __hash__ = .__ hash__ Define Do not want to execute hash __hash__ = None. Increase the TypeError exception if trying to redeploy the hash functionExample 3: hash () for custom objects by overriding __hash __ ()
class Person: def __init__(self, age, name): self.age = age self.name = name def __eq__(self, other): return self.age == other.age and self.name == other.name def __hash__(self): print('Giá trị hash là:') return hash((self.age, self.name)) TipsMake = Person(23, 'Adam') print(hash(TipsMake))
When running the program, the result returned is:
Giá trị hash là: -7852579163592371862
Note: You do not need to execute the __eq __ () function for the hash process because this function is implemented by default for all objects.
You should read it
- What is hash? Hash application
- What is Cryptographic Hash (cryptographic hash function)?
- Max () function in Python
- The ord () function in Python
- Zip () function in Python
- Int () function in Python
- The function id () in Python
- The oct () function in Python
- The next () function in Python
- Hex () function in Python
- The map () function in Python
- The function dir () in Python
Maybe you are interested
What are hashtags? How to use hashtags and their uses?
Latest Code Will of Hashira
How to hash and verify passwords in Node.js using bcrypt
List of file names, HASH SHA-256 codes containing WannaCry malware
How to fix status_invalid_image_hash error on Microsoft Edge
How to create a torrent magnet link with info hash