Strptime () function in Python
In this article, TipsMake.com will show you how to create a datetime object (date, time, and time) from the corresponding string with specific examples to make it easier to visualize and capture the function.
The strptime () function in Python is used to create the datetime object from a given string. However, not any string can be passed into the function, the string here must satisfy a certain format to return the result.
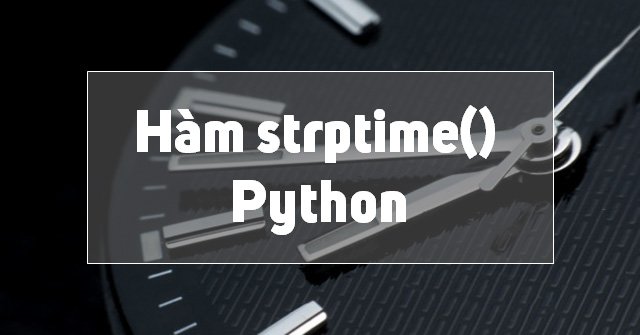
Example 1: Convert string to datetime object
from datetime import datetime date_string = "11 July, 2019" print("date_string =", date_string) date_object = datetime.strptime(date_string, "%d %B, %Y") print("date_object =", date_object)
Run the program, the result returned:
date_string = 11 July, 2019 date_object = 2019-07-11 00:00:00
How does strptime () work?
Strptime () has two parameters:
- The string will be converted to datetime.
- Code format .
Based on the string and format code passed, the method returns its corresponding datetime object.
In the above example:
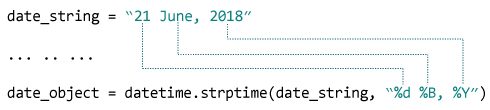
%d
: Representing the day of the month. For example: 01, 02, ., 31.%B
: Full month name. Example: January, February .%Y
: Four-digit year. Examples: 2018, 2019 .
Example 2: Convert string to datetime object
from datetime import datetime dt_string = "11/07/2018 09:15:32" # Định dạng ở dạng dd/mm/yyyy dt_object1 = datetime.strptime(dt_string, "%d/%m/%Y %H:%M:%S") print("dt_object1 =", dt_object1) # Định dạng ở dạng mm/dd/yyyy dt_object2 = datetime.strptime(dt_string, "%m/%d/%Y %H:%M:%S") print("dt_object2 =", dt_object2)
Run the program, the result returned:
dt_object1 = 2018-07-11 09:15:32 dt_object2 = 2018-11-07 09:15:32
List of code formats
The table below shows all the formatting codes that you can pass to the strptime () method.
%a
Abbreviated name of the week Sun, Mon . %A
The day of the week is written in full Sunday, Monday . %w
Day of the week, numeric values 0, 1, ., 6 %d
Date of the month, numeric value form (value 0 as a buffer before the 1-digit date) 01, 02, ., 31 %-d
Day of the month, value form 1, 2, ., 30 %b
Abbreviated month name Jan, Feb, ., Dec %B
Full month name, January, February . %m
Month of the year, numeric value (value 0 as a buffer before the month has 1 digits) 01, 02, ., 12 %-m
Month of the year, value form 1, 2, ., 12 %y
2-digit year value (with value 0 as buffer before the year has 1 digit) 00, 01, ., 99 %-y
Year 2 digit values 0, 1, ., 99 %Y
Full year value 2013, 2019 . %H
Hourly system 24 language (value 0 as a buffer before 1 digit) 00, 01, ., 23 %-H
24 hour system time, format values of 0, 1, ., 23 %I
12 hour system time, numeric value (value 0 as a buffer with 1 digit before) 01, 02, ., 12 %-I
12 hour system hours 1, 2, ., 12 %p
Local time is AM or PM. AM, PM %M
Minutes, numeric values (value 0 as 1-minute pre-minute buffers) 00, 01, ., 59 %-M
Minutes, numeric values 0, 1, . , 59 %S
Seconds, numeric values (value 0 as a buffer before the 1-digit second) 00, 01, ., 59 %-S
Seconds, numeric values 0, 1, ., 59 %f
Micro seconds, numeric value (value 0 as a 1-second pre-pad buffer) 000000 - 999999 %z
UTC compensation time in + HHMM or -HHMM format. %Z
Time zone name %j
Date of the year, numeric value (value 0, 00 as a buffer before the date has 1 and 2 digits) 001, 002, ., 366 %-j
Year of the year, form values of 1, 2, ., 366 %U
Number of weeks in the year (Sunday is the first day of the week). All days in the new year before the first Sunday are considered as during the week of 00, 01, ., 53 %W
Number of weeks in the year (Monday is the first day of the week). All days in the new year before the first Monday are considered in week 0. 00, 01, ., 53 %c
Returns the date and time Sep 30 30:06:05 2013 %x
Returns on 09 / 30/13 %X
Returns 7:06:05 %%
'%' character literally. % ValueError results returned from strptime ()
If the two string parameters and the format code passed into strptime () do not match, you will get the ValueError result .
from datetime import datetime date_string = "11/07/2018" date_object = datetime.strptime(date_string, "%d %m %Y") print("date_object =", date_object)
Results returned:
ValueError: time data '11/07/2018' does not match format '%d %m %Y'
Previous lesson: strftime () function in Python
Next lesson: The current date and time in Python
You should read it
- Datetime in Python
- Strftime () function in Python
- Current date and time in Python
- Module time in Python
- Convert the timestamp value in Python
- Function sleep () in Python
- Function handles DATE / TIME in SQL - Part 1
- The oct () function in Python
- The map () function in Python
- The next () function in Python
- The function set () in Python
- Max () function in Python